What's a delegate
A delegate is a type that safely wraps/encapsulate a method. It is similar to 'function pointers' in C/C++, but it's type safe.
When to use a delegate
Use a delegate when :
- An eventing design pattern is used.
- It is desirable to encapsulate a static method.
- The caller has no need access other properties, methods, or interfaces on the object implementing the method.
- Easy composition is desired.
- A class may need more than one implementation of the method.
There was a good example given on a book that I recently referenced.
'Consider your will—your last will and testament. It’s a set of instructions—“pay the bills, make a donation to charity, leave the rest of my estate to the cat,” for instance. You write it before your death, and leave it in an appropriately safe place. After your death, your attorney will (you hope!) act on those instructions.'
In order to a delegate to proceed, following four things need to happen:
* The delegate type needs to be declared.
* There must be a method containing the code to execute.
* A delegate instance must be created.
* The delegate instance must be invoked.
Declaring delegate type
Declaration of a delegate type specifies what kind of action can be represented by instances of the type.
E.g :
delegate void MySampleDelegate(string zParameter)
If we are creating an instance of the above delegate, we should have a method with one string parameter and void return type.
Method containing the code to execute
There should be a method which matches the signature of the delegate. Method can be either static or non static. I will take two examples to illustrate a static method and a non static method. I will use the following two methods in the 'DelegateClass' class.
E.g :
public class DelegateClass {
public static void MySampleDelegateMethodStatic(string zParameter) {
Console.WriteLine("From static method. Parameter : {0}", zParameter);
}
void MySampleDelegateMethodNonStatic(string zParameter) {
Console.WriteLine("From non static method. Parameter : {0}", zParameter);
}
}
Creating a delegate instance
When creating delegate instances static methods can be passed directly. But for non static methods an instance should be created and passed that to the delegate.
E.g : [Static Method]
MySampleDelegate delMethodA = new MySampleDelegate(DelegateClass.MySampleDelegateMethodStatic);
E.g : [Non Static Method]
DelegateClass deligateClass = new DelegateClass();
MySampleDelegate delMethodB = new MySampleDelegate(deligateClass.MySampleDelegateMethodNonStatic);
Or
MySampleDelegate delMethodB = new MySampleDelegate(new DelegateClass().MySampleDelegateMethodNonStatic);
Invoking delegate method
It's a matter of calling the Invoke method on the delegate instance.
E.g :
delMethodA.Invoke("Parameter A");
delMethodB.Invoke("Parameter B");
Here is the complete source code for the above example. I have used a console application to illustrate this :
using System;
using System.Text;
namespace SampleConsoleApplicationA {
delegate void MySampleDelegate(string zParameter);
class Program {
static void Main(string[] args) {
MySampleDelegate delMethodA = new MySampleDelegate(DelegateClass.MySampleDelegateMethodStatic);
MySampleDelegate delMethodB = new MySampleDelegate(new DelegateClass().MySampleDelegateMethodNonStatic);
delMethodA.Invoke("Parameter A");
delMethodB.Invoke("Parameter B");
Console.ReadLine();
}
}
public class DelegateClass {
public static void MySampleDelegateMethodStatic(string zParameter) {
Console.WriteLine("From static method. Parameter : {0}", zParameter);
}
public void MySampleDelegateMethodNonStatic(string zParameter) {
Console.WriteLine("From non static method. Parameter : {0}", zParameter);
}
}
}
And when you run your application you will get the following output:
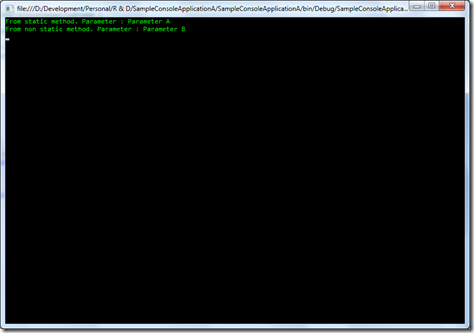
Instead of using separate delegate instances, you can combine and execute delegates. We have to use the 'Combine' method in Delegate class. To illustrate this I will alter my 'DelegateClass' as shown below :
public class DelegateClass {
public void MySampleMethodA(string zParameter) {
Console.WriteLine("MySampleMethodA. Parameter : {0}", zParameter);
}
public void MySampleMethodB(string zParameter) {
Console.WriteLine("MySampleMethodB. Parameter : {0}", zParameter);
}
public void MySampleMethodC(string zParameter) {
Console.WriteLine("MySampleMethodC. Parameter : {0}", zParameter);
}
}
To create instances and invoke :
MySampleDelegate[] mySampleDelegates = new MySampleDelegate[]{
new MySampleDelegate(new DelegateClass().MySampleMethodA),
new MySampleDelegate(new DelegateClass().MySampleMethodB),
new MySampleDelegate(new DelegateClass().MySampleMethodC)
};
MySampleDelegate sample = (MySampleDelegate)Delegate.Combine(mySampleDelegates);
sample.Invoke("Parameter X");
Or
MySampleDelegate D, deligateA, deligateB, deligateC;
deligateA = new DelegateClass().MySampleMethodA;
deligateB = new DelegateClass().MySampleMethodB;
deligateC = new DelegateClass().MySampleMethodC;
D = deligateA + deligateB + deligateC;
D("Parameter X");
The complete source code :
using System;
using System.Text;
namespace SampleConsoleApplicationA {
delegate void MySampleDelegate(string zParameter);
class Program {
static void Main(string[] args) {
MySampleDelegate[] mySampleDelegates = new MySampleDelegate[]{
new MySampleDelegate(new DelegateClass().MySampleMethodA),
new MySampleDelegate(new DelegateClass().MySampleMethodB),
new MySampleDelegate(new DelegateClass().MySampleMethodC)
};
MySampleDelegate sample = (MySampleDelegate)Delegate.Combine(mySampleDelegates);
sample.Invoke("Parameter X");
/*
MySampleDelegate D, deligateA, deligateB, deligateC;
deligateA = new DelegateClass().MySampleMethodA;
deligateB = new DelegateClass().MySampleMethodB;
deligateC = new DelegateClass().MySampleMethodC;
D = deligateA + deligateB + deligateC;
D("Parameter X");
*/
Console.ReadLine();
}
}
public class DelegateClass {
public void MySampleMethodA(string zParameter) {
Console.WriteLine("MySampleMethodA. Parameter : {0}", zParameter);
}
public void MySampleMethodB(string zParameter) {
Console.WriteLine("MySampleMethodB. Parameter : {0}", zParameter);
}
public void MySampleMethodC(string zParameter) {
Console.WriteLine("MySampleMethodC. Parameter : {0}", zParameter);
}
}
}
An when you run the application, you will get the following output:
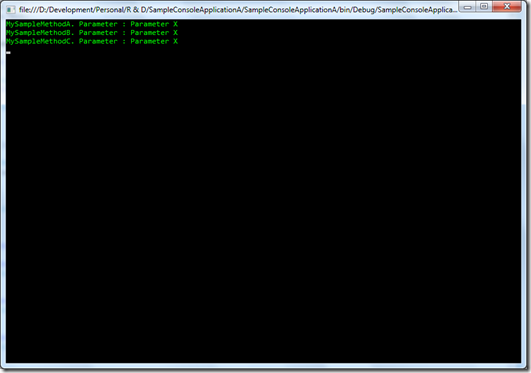